Let’s make python RSS reader using Django backend framework. You can consider it a mini project idea for computer science and IT students
RSS stands for Real Simple Syndication or Rich Site Summary. Here we are going to make a simple RSS reader using python and django framework. Computer science or IT students can make a mini project on this.
What are we going to do exactly?
We are going to develop a python RSS reader in that we will create a web page interface that accepts external RSS URL and gives output on a web page in a very simple manner (RSS feed parser). The output will be headlines of the news, a small summary or description of that news, and a link to the specific news.
5 Best reference books for metallurgy
For RSS reader, we are going to use a powerful backend python framework – Django. In the frontend, we are going to use simple CDN bootstrapping.
I’m using VS code as a code edit for this tutorial. You are free to use any of your favorite code editors.
In case you need to host this project online then you can check the best hosting for India. It will help you to share your project with your friends or teachers.
Python RSS feed parser
Let’s create a folder and name it as a RSSReader (You can name it anything). Now open your code editor and open a created folder in the code editor. Create a virtual environment to keep project files isolated from your other computer files. For this, type the following command in the terminal:
Now activate the virtual environment using the following command:
mysiteScriptsactivate
Let’s install django framework using the following command:
pip install django
Also, install the feedparser python library for parsing RSS feeds:
pip install feedparser
Now create a project in django with the name “rssreader”
django-admin startproject rssreader .
Create a new app with the name “rss” using:
python manage.py startapp rss
Go to the settings.py file and add app name ‘rss’ in the INSTALLED_APPS =[] as shown below:
INSTALLED_APPS = [
‘django.contrib.admin’,
‘django.contrib.auth’,
‘django.contrib.contenttypes’,
‘django.contrib.sessions’,
‘django.contrib.messages’,
‘django.contrib.staticfiles’,
‘rss’,
]
Create a “templates” folder inside the rss folder like “rss/templates”. Again create a folder “rss” folder inside the templates folder. It should look like: “rss/templates/rss” . Now create reader.html and base.html files this rss folder.
In reader.html, type the following code:
reader.html
{% extends ‘rss/base.html’ %}
{% block body %}
<br />
<form class=“form-inline”>
<div class=“form-group mx-sm-3 mb-2”>
<label for=“inputPassword2” class=“sr-only”>Password</label>
<input type=“text” class=“form-control” placeholder=“paste link”
name=“url”>
</div>
<button type=“submit” class=“btn btn-primary mb-2”>Search</button>
</form>
{% if feed %}
<h2>{{ feed.feed.title }}</h2>
{% if feed.entries %}
{% for entry in feed.entries %}
<div class=“card”>
<div class=“card-header”>
Feed
</div>
<div class=“card-body”>
<h5 class=“card-title”>Headline: {{ entry.title }}</h5>
<p class=“card-text”>Description: {{ entry.description }}</p>
<a href=“{{ entry.link }}” class=“btn btn-primary”>Visit Link</a>
</div>
</div><p>{{ entry.published }}</p>
{% endfor %}
{% endif %}
{% else %}
<br />
<p>Enter your favorite RSS feed above.</p>
{% endif %}
{{ feed2 }}
{% endblock %}
In base.html type the following code:
base.html
<!DOCTYPE html>
<head>
<title>Django RSS Reader</title>
</head>
<nav class=“navbar navbar-expand-sm bg-dark navbar-dark”>
<ul class=“navbar-nav”>
<li class=“nav-item active”>
<a class=“nav-link” href=“/”>Home</a>
</ul>
</nav>
<body>
{% block body %}{% endblock %}
</body>
</html>
For cdn bootstrapping, add below lines before </head> in base.html
<script src=”https://code.jquery.com/jquery-3.3.1.slim.min.js”></script>
<script src=”https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.3/umd/popper.min.js”></script>
<script src=”https://stackpath.bootstrapcdn.com/bootstrap/4.1.2/js/bootstrap.min.js”></script>
<link rel=”stylesheet” href=”https://stackpath.bootstrapcdn.com/bootstrap/4.1.2/css/bootstrap.min.css”>
We are done with the template formatting. Now to go views.py file in the rss app folder and type the following code in it. Remove all previous code.
Views.py
from django.shortcuts import render
from django.http import HttpResponse
import feedparser
def index(request):
if request.GET.get(“url”):
url = request.GET[“url”] #Getting URL
feed = feedparser.parse(url) #Parsing XML data
else:
feed = None
return render(request, ‘rss/reader.html’, {
‘feed’ : feed,
})
Now open urls.py file from main project folder “rssreader”. Its location should look like “rssreader/urls.py” . Remove all previous code from the file and type following code:
rssreader/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path(‘admin/’, admin.site.urls),
path(”, include(‘rss.urls’)),
]
Its time to create url route for your app. For this, create urls.py file in rss app folder. Its location should look like “rss/urls.py”. Type the following code in that file. Don’t forget to remove default code from the file.
rss/urls.py
from django.urls import path, include
from . import views
urlpatterns= [
path(”, views.index, name=‘index’)
]
It’s time to run our RSS reader Django project in the browser. Type the following command in the terminal:
python manage.py runserver
After its successful execution in the terminal open any web browser and go to this address http://127.0.0.1:8000/ Here you can see your RSS reader webpage.
For testing, type RSS feed url of BBC news website in the search box
URL : http://feeds.bbci.co.uk/news/rss.xml
Now you can see live news feeds from the BBC website on your RSS reader webpage.
You can see the screenshot of RSS reader webpage:
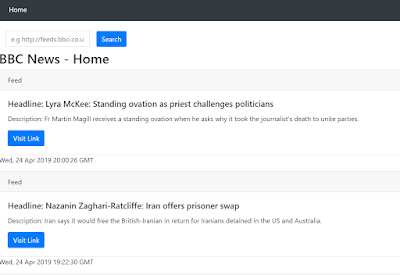 |
RSS reader using python and django |
To host this project on hosting websites, check out
hosting discount to get discount on hosting and save some money.
If you are facing any problem regarding python RSS reader then feel free to comment below. I will try my best to solve your problem
can we add another RSS feed instead of BBC??
yes
Can we add another RSS with Kinect
Thank you sharing such good information. I had one suggestion for CSE Projects